Install
- Apache
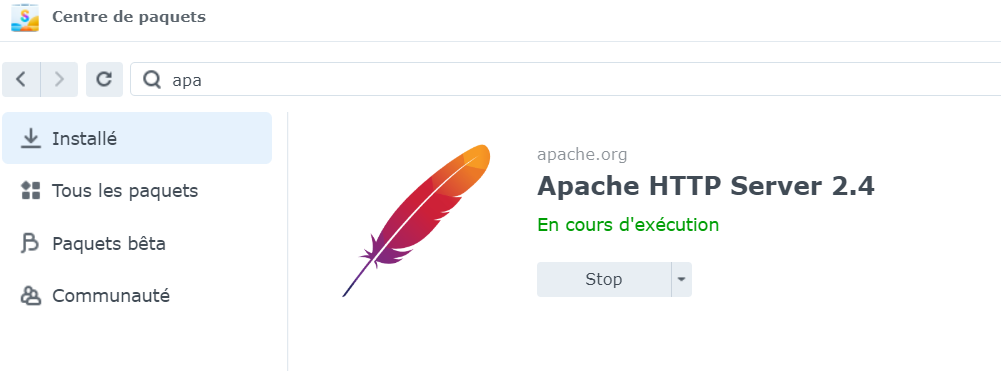
- WebStation
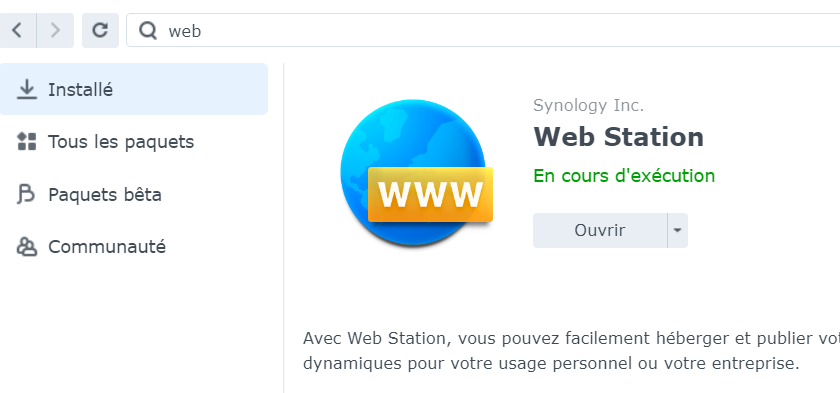
- PHP
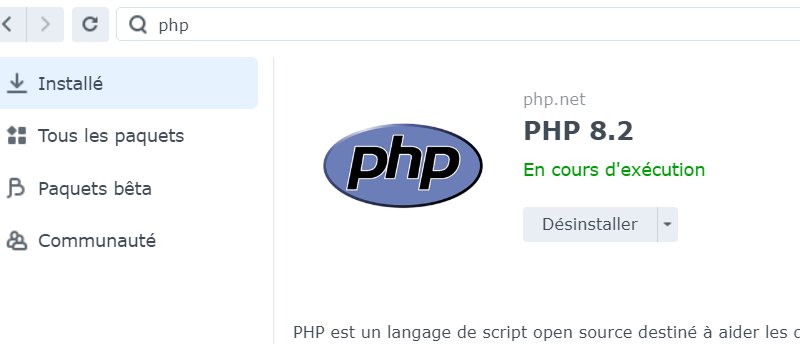
PHP Profile
On Web station
- Create a new php profile
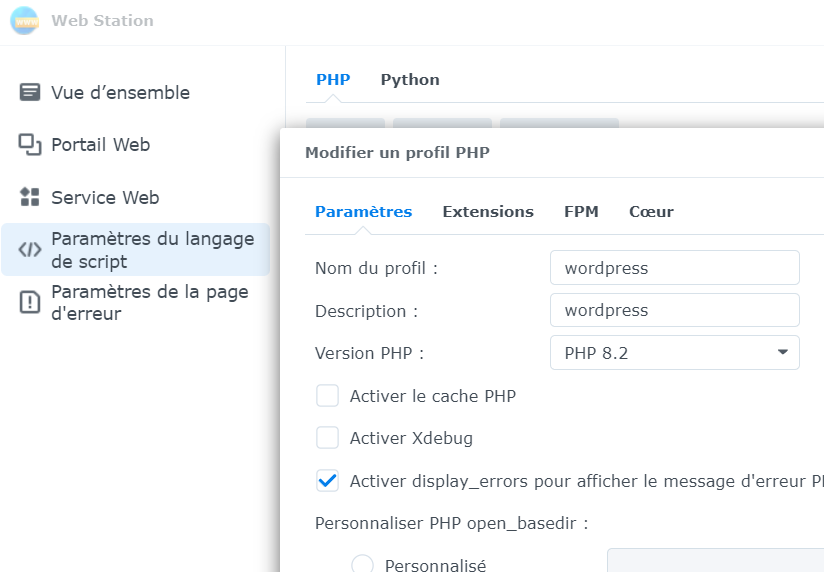
- I used an existing PHP configuration with all features enabled
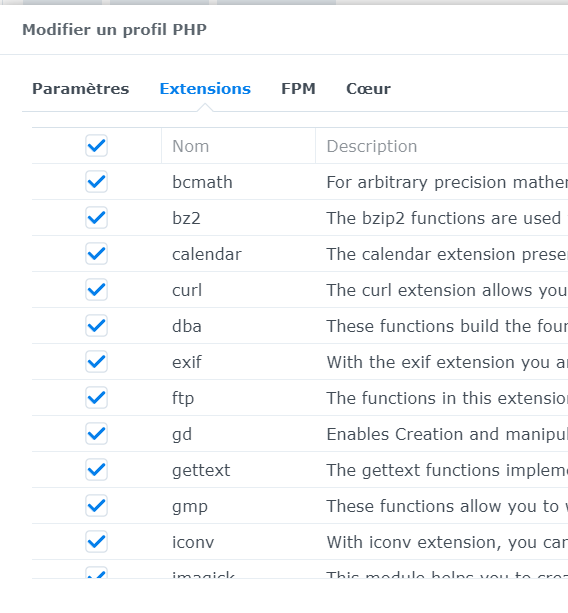
New Web service
On web Station
- Create a new web station using your PHP profile
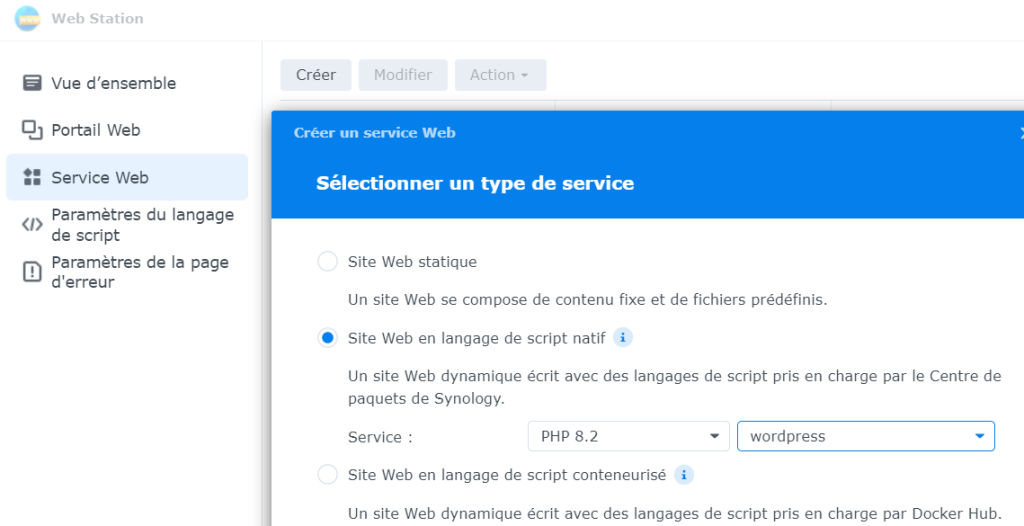
- Select Name and directory
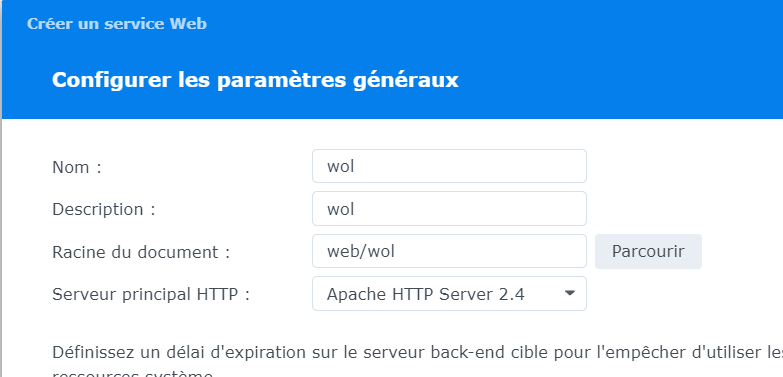
Create file
Create two files with the same name in the web directory :
- Index.html
Change button onclick
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Wake-on-LAN</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
function sendWakeOnLan(macAddress) {
$.ajax({
type: "POST",
url: "wol.php", // Assurez-vous que cette URL est correcte
data: { mac: macAddress },
success: function(response) {
alert(response);
},
error: function() {
alert("Error sending the magic packet.");
}
});
}
</script>
<style>
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
.button-container {
display: flex;
justify-content: space-around;
width: 100%;
max-width: 600px; /* Vous pouvez ajuster cette largeur selon vos besoins */
}
button {
font-size: 16px;
padding: 10px 20px;
color: white;
background-color: #007BFF;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s, transform 0.2s;
}
button:hover {
background-color: #0056b3;
transform: scale(1.05);
}
button:active {
transform: scale(0.95);
}
</style>
</head>
<body>
<div class="button-container">
<button onclick="sendWakeOnLan('00:CC:91:AA:72:DA')">Computer3</button>
<button onclick="sendWakeOnLan('00:C6:91:AF:3D:00')">Computer4</button>
</div>
</body>
</html>
- wol.php
Change 192.168.0.255 by your subnet network
<?php
function wakeOnLan($broadcastAddress, $macAddress) {
$macAddress = str_replace([':', '-'], '', $macAddress);
$magicPacket = str_repeat(chr(255), 6) . str_repeat(pack('H12', $macAddress), 16);
if (!($sock = socket_create(AF_INET, SOCK_DGRAM, SOL_UDP))) {
return "Cannot create socket";
}
$options = socket_set_option($sock, SOL_SOCKET, SO_BROADCAST, 1);
if (!$options) {
socket_close($sock);
return "Failed to set socket option";
}
$send = socket_sendto($sock, $magicPacket, strlen($magicPacket), 0, $broadcastAddress, 9);
socket_close($sock);
if (!$send) {
return "Failed to send magic packet";
}
return "Wake-on-LAN packet has been sent to $macAddress.";
}
if ($_SERVER['REQUEST_METHOD'] === 'POST' && !empty($_POST['mac'])) {
echo wakeOnLan('192.168.0.255', $_POST['mac']);
} else {
echo "Invalid request";
}
?>
Result
On your web site https://synologyserver/wol
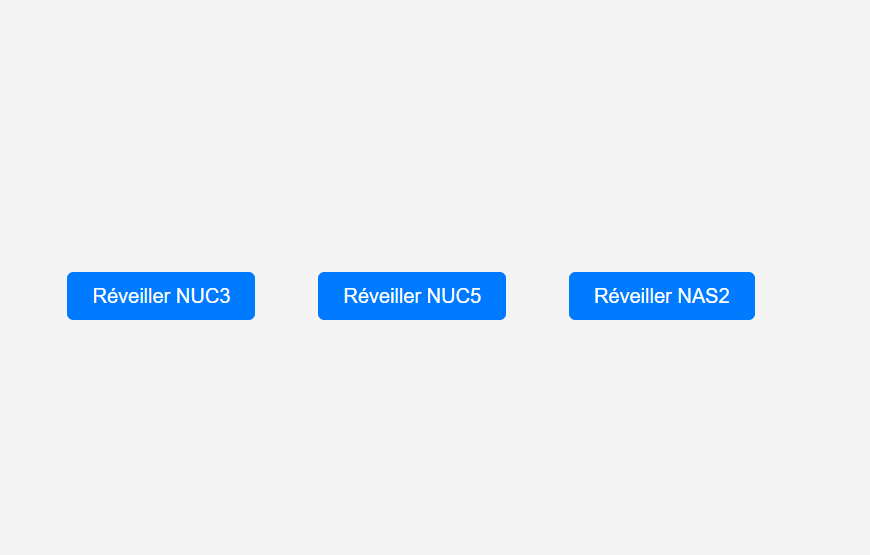
https://github.com/DavidWuibaille/Repository/tree/main/Synology/WOL
0 Comments